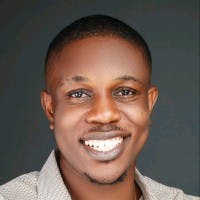
Available for Work
The Definitive Guide to Using Axios for Data Fetching in React
Explore the comprehensive guide on using Axios for data fetching in React. From installation to advanced techniques, learn to seamlessly integrate API requests, ensuring dynamic and responsive applications
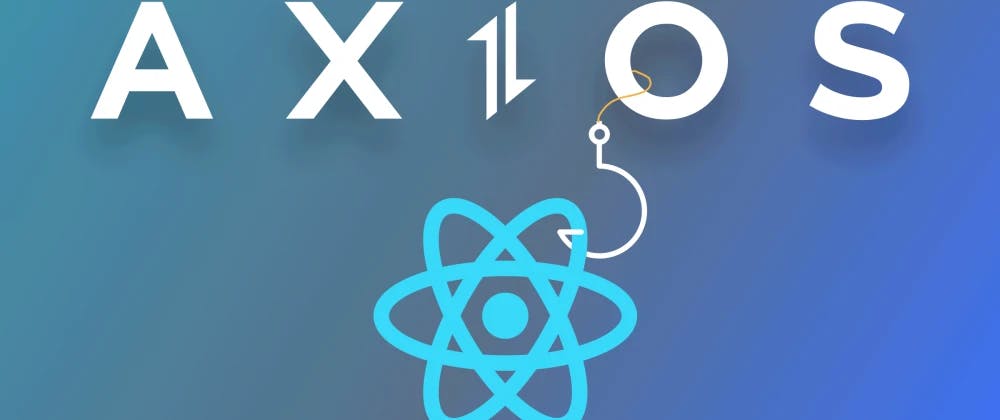
A Guide to Using Axios for Data Fetching in React
When it comes to building dynamic and interactive web applications, fetching data from APIs is a common requirement. React, a popular JavaScript library for building user interfaces, provides a straightforward way to handle data fetching through various methods. One widely used library for making HTTP requests in React applications is Axios. In this guide, we'll explore how to use Axios for data fetching in React.
What is Axios?
Axios is a promise-based HTTP client for the browser and Node.js. It is known for its simplicity and flexibility, making it a popular choice for handling asynchronous operations, such as making API requests in React applications.
Installing Axios
To get started, you need to install Axios in your React project. Open your terminal and run the following command:
npm install axios
Or, if you prefer using Yarn:
yarn add axios
This will add Axios to your project's dependencies.
Importing Axios in Your Component
Once Axios is installed, you can import it into your React component where you plan to make API requests. Add the following import statement at the top of your file:
import axios from 'axios';
Making a Simple GET Request
Let's start with a basic example of making a GET request to retrieve data from an API. In this example, we'll use the axios.get()
method. Suppose you want to fetch data from the JSONPlaceholder API:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts');
setData(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Data from API:</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.title}</li>
))}
</ul>
</div>
);
};
export default MyComponent;
In this example, the useEffect
hook is used to trigger the data fetching when the component mounts. The axios.get()
method returns a promise, and await
is used to handle the asynchronous nature of the request. The retrieved data is then stored in the component's state using the setData
function.
Handling POST Requests
Axios also supports other HTTP methods, such as POST, PUT, and DELETE. Here's an example of making a POST request to send data to an API:
import React, { useState } from 'react';
const MyPostComponent = () => {
const [postData, setPostData] = useState({ title: '', body: '' });
const handlePost = async () => {
try {
const response = await axios.post('https://jsonplaceholder.typicode.com/posts', postData);
console.log('Post successful:', response.data);
} catch (error) {
console.error('Error posting data:', error);
}
};
return (
<div>
<h1>Post Data to API:</h1>
<form onSubmit={(e) => {
e.preventDefault();
handlePost();
}}>
<label>Title:
<input type="text" value={postData.title} onChange={(e) => setPostData({ ...postData, title: e.target.value })} />
</label>
<br />
<label>Body:
<textarea value={postData.body} onChange={(e) => setPostData({ ...postData, body: e.target.value })}></textarea>
</label>
<br />
<button type="submit">Post Data</button>
</form>
</div>
);
};
export default MyPostComponent;
In this example, the axios.post()
method is used to send a POST request with the data provided in the postData
state.
Conclusion
Axios simplifies the process of making HTTP requests in React applications. Whether you need to fetch data from an API or send data to it, Axios provides an easy-to-use interface. By incorporating Axios into your React project, you can efficiently manage asynchronous operations, making your application more dynamic and responsive. Experiment with different Axios methods and explore its extensive features to enhance your data fetching capabilities in React.
Lagos, Nigeria
More about Me

SEP 7, 2024
From Curiosity to Impact: The Proven Path to Lasting Motivation and Success
Learn More

AUG 31, 2024
Unlocking Creativity: The Secrets to Unleashing Your Potential
Learn More

AUG 24, 2024
The Science Behind Achieving the Impossible: Unveiling the Formula for Success.
Learn More

AUG 4, 2024
How Walking Transformed My Life (13k+ Steps A Day Changed My Life)
Learn More

JUL 30, 2024
From Ordinary to Extraordinary: How Health Can Revolutionize Every Aspect of Your Life
Learn More
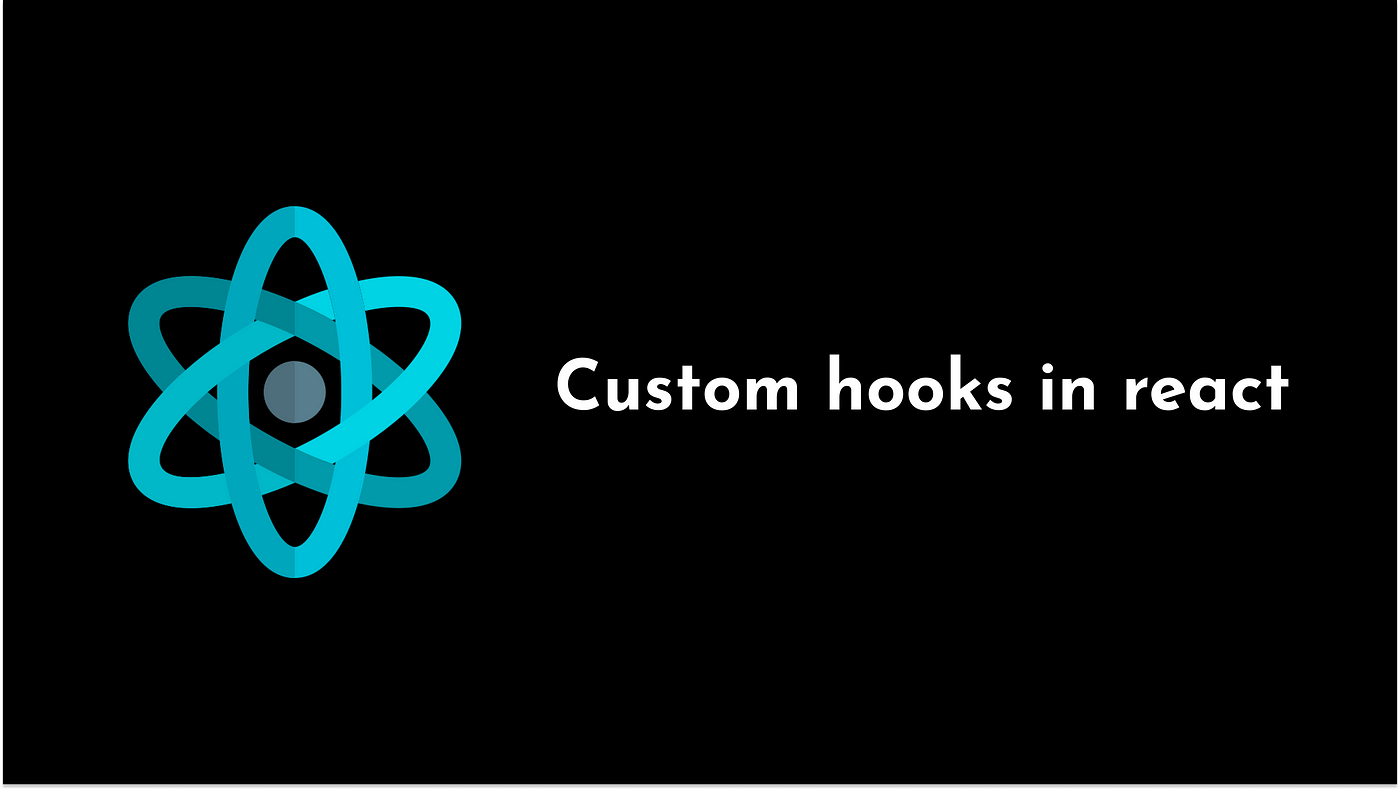